일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | ||||
4 | 5 | 6 | 7 | 8 | 9 | 10 |
11 | 12 | 13 | 14 | 15 | 16 | 17 |
18 | 19 | 20 | 21 | 22 | 23 | 24 |
25 | 26 | 27 | 28 | 29 | 30 | 31 |
- SQL
- 태블로 신병훈련소 후기
- 태블로 신병 훈련소 후기
- python udemy
- 태블로신병훈련소
- 태블로 신병 훈련소 10기
- 알약 모양
- TABLEAU Certificate
- Python
- 태블로 자격시험
- 데이터 시각화
- 태블로 신병 훈련소
- coursera
- 태블로 독학
- tableau
- 태블로
- 태블로 신병훈련소
- 태블로 자격증 독학
- 태블로 리뷰
- 태블로 자격시험 독학
- 태블로 신병 훈련소 11기
- 태블로초보
- 태블로독학
- 태블로 데스크탑
- 범프차트
- 태블로 집합
- CourseraSQL
- 태블로 씹어먹기
- 빅데이터분석기사
- 태블로 무료 강의
- Today
- Total
하루에 하나씩
Python basic - Function & Method 본문
Function & Custom function
Creating function
- def : creating function
- For executing a function, have those open and close parentheses ().
Adding parameters to a function
- function을 실행시킬 때, ()안에 argument를 넣지 않으면 error가 발생함
오류 내용 : name에 parameter와 짝지어지는 posintional argment 누락
* Positional argument란, 인자를 정의된 매개변수의 순서대로 전달하는 방법
def add_and_dev(a,b,c)
print(f"인자 전달 순서 : {a}, {b}, {c}")
result = (a+b)/c
return print(f"결과값:{Result}")
add_and_dev(5, 10, 15)
* 반대로 Keyword argument는 매개변수를 지정하여 인자를 전달하는 방법 (헷갈리지 않고 원하는 결과를 유도 할 수 있음)
add_and_dev(c=15, a= 5, b= 10)
Default argument
- default parameter 또는 default argment 값을 지정하고 싶다면, fuction을 생성할 때 ()안에 equal(=) to '값' 지정함
- 이 경우 argument를 누락하게 되어도 error가 발생하지 않고, 미리 지정해둔 default 인자가 배정됨
Quick Tip
Docstring(주석) 생성: Three ' or Three "
주속 보기: shift + tab
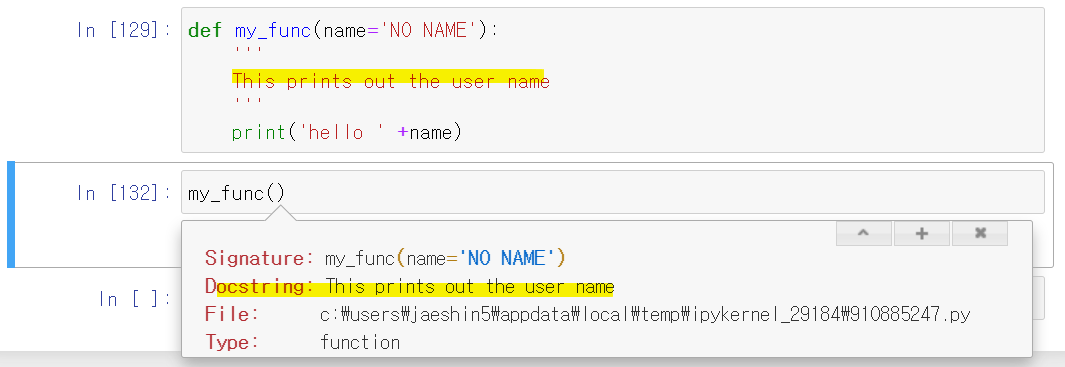
print vs return
print: just displays the actual value
returns: save the actual object
- return keyword can save this as a variable
(Run result it returns the value that was saved before)
result값에 square(2) 실행한 값을 저장만하고 출력하지 않음. 그러나 사후에 result를 실행하면 result에 저장된 sqaure(2) 결과 즉, 4 값을 출력시킨다
- the print keyword only prints out the value (save x)
result = square(2)를 실행하면 4 값을 출력하지만 result를 실행하면 아무 값도 나오지 않음
The way I can do the assignment to a new variable is by using the return keyword
Lamda Expression
Lambda expression (=can quickly type in anonymous function)
If you only plan on using a function one time, and maybe you don't want to rewrite the entire- Lambda keyword/ input/ colon/ output
- How to convert the function to Lambda
1) take away the name
2) take away def keyword and replace it with lambda
3) take away parentheses
4) take away return keyword
def keyword를 사용해 함수를 정의하는 방법
Lamda식으로 함수를 쓰는 방법
Methods on strings
Upper, Lower
Split
- Split the string into a list based on the white space.
- Split on a particular string value → tweet.split('#')
* now the hash tag is no longer in this list because that is what was split on.
- 응용: list Negative -1으로 hash tag가 달린 마지막 string만 출력하는 법
keys and items of a dictionary
.keys() / .items()
Grab the keys and items of a dictionary
Dictionaries do not retain any order.
.pop()
How you can pop something off of a list or check if something is inside of a list
사용법 :
If I want to grab and remove the last item in a list
(missing the last item of the list -> save it the valuable last so that they can remove an item from a list)
.pop(index number)
You can just pass that into pop if you want to remove an item at a specific index.
Inner operator
- in [ , , ] / in list_name
If I want to check if an item is inside of a list, I can just use the inner operator.
'Python' 카테고리의 다른 글
Section 3: Machine Learning Pathway Overview (0) | 2023.10.03 |
---|---|
Python Basic - 02-Python Crash Course Exercises (0) | 2023.10.01 |
Python Basic - Comparison operator & Logic operator (0) | 2023.08.14 |
Python basic - .format & List & Tuples & Dictionaries (0) | 2023.08.13 |
[Python] 2. Expressions (0) | 2023.01.03 |